CanJS has everything you need to build a web app’s front-end: components, templating, models, and more. We also publish all of the modules that provide the infrastructure for the higher-level APIs, including a utility library called can-util.
Subscribe
Get our best content on how to build better apps.
Subscribe
Get our best content on how to build better apps.
Got product development questions?
Join us on
Discord
Building a Real-time, Multi-platform App in 3 Months
Bitovi worked with HaulHound, a logistics technology company, to design and develop a system connecting truckers and shippers. The system we built supports real-time data, an iOS and Android app for truckers, and a web application for shippers.
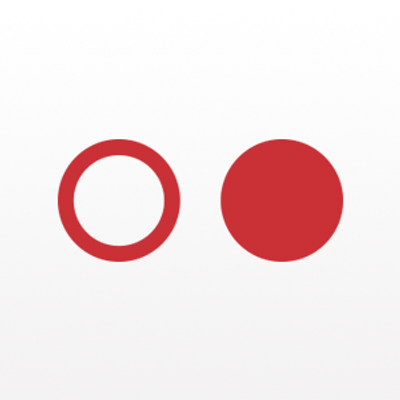
The Bitovi Team
How To Conditionally Load Modules with StealJS
StealJS makes it easy to bundle all of your JavaScript modules into highly efficient production builds, but sometimes you don’t want to bundle everything together. There are times when a module only needs to be loaded when certain conditions are met; it might be a polyfill for a browser without support for a new API or a translation file based on the user’s preferred language.
Manuel Mujica
Module Loaders: Master the Pipeline!
We will focus on how a JavaScript module can be loaded, and get a gist of what all module loaders do.
If you are new to modules in JavaScript I would recommend to start with this article by Addy Osmani.
Do you use Browserify, WebPack, jspm, StealJS, or SystemJS? This article will provide a peak under the hood at the layer on top of which those libraries are implemented.
The nature of a human brain is that it cannot deal with a lot of objects at the same time (Miller’s Law). If you are going to build a large JavaScript application, you should stop, remember this limitation and start thinking in terms of modules.
Modules are a way to organize your application. Simply break your functionality into small pieces, focusing on how they will work with each other, and then assemble them together. A module could be seen as a black box with a clear, simple API. Modules commonly depend on other modules.
In today's modern browsers, there is support for not only writing and loading modules, but performing various low-level tasks around loading and executing the module. This article will explain the current standard for module loaders - their lifecycle and their hooks. A future article will show a detailed example for how to use these hooks.
Pop quiz! If you have modules written in CoffeeScript and CommonJS, is it possible to use them both within an ES6 application?
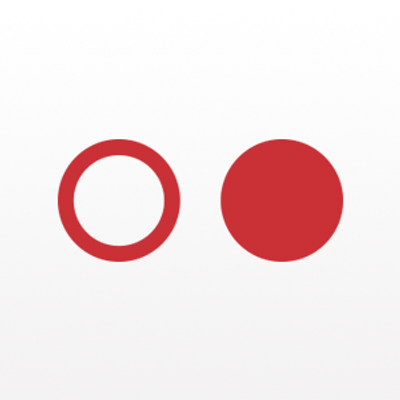
The Bitovi Team
Simplify your Unit Tests with Dependency Injection for JavaScript Modules
Note: Come join us for a live training on Dependency Injection with StealJS on Wednesday, March 16, 2016 at 12:30 PM CST.
Writing small modules with good unit tests greatly increases the maintainability of your code. However, it can be difficult to test a module without first understanding how its dependencies work. Dependency Injection (DI) allows you to isolate your tests to exactly what you’re trying to test.
While many Dependency Injection implementations require you to use them as plumbing throughout your code, you can use Dependency Injection with the JavaScript module format you’re already using by loading your modules with StealJS. No need to modify your code -- use Dependency Injection when you want it and your existing module format to load modules everywhere else.
What is Dependency Injection?
Dependency injection is a Computer Science design principle that has been around a long time. There are lots of articles (here are a few) that go in depth on this topic, but I’ll use this simple definition:
Dependency Injection - replacing the code that will be loaded when a JavaScript module is imported.
The most useful way to use DI in JavaScript is to simplify unit testing. To better understand this, we'll go over the simple example shown in the GIF above.
Take these two modules:
user
- exports aname
function that makes a call to the server and returns the current user’s name.navigation
- exports agreeting
function that uses theuser.name
function to display “Welcome Back, name!” if we know the user’s name or just “Welcome!” if we don’t.
In the next sections, we'll show how to unit test them, with and without using DI.
Testing Without Dependency Injection
If you want to test navigation.greeting
without Dependency Injection, you need user.name
to return a value. One way to do this is to intercept the request being made to the server and return a mocked AJAX response with the data needed for your test case.
With this approach, you’re testing more than just the greeting
function:
In order to set this up you also have to know implementation details about the user
module.
- What request is it making to the server?
- Is it using a library or framework to make the request?
- What does the server’s response look like?
If the user
module changes, you might have to modify your test, even if the changes don’t affect the navigation
module. Consider the following scenarios:
What happens if the server’s response changes?
What happens if the user
module starts caching its data in localStorage?
The paradigm of unit testing is broken. Unit testing should be isolating and testing a single module, but in practice, this unit test is dependent on many modules that we’re not trying to test.
The tight coupling of the navigation
module’s tests to the implementation of the user
module leads to brittle tests and lots of frustration - if you don’t just give up on testing the module altogether.
Testing With Dependency Injection
Testing the navigation
module becomes much easier when using dependency injection because you can inject a version of the user
module that does exactly what you need for your navigation
module’s tests:
Now if the implementation of user.name
changes, it won’t affect your tests at all.
Dependency Injection with StealJS
StealJS is a client-side JavaScript module loader. A recent extension to steal, steal-clone, added the ability to do dependency injection of JavaScript modules. Here is the navigation
example using steal-clone:
Navigation Module Code:
import { name } from 'models/user';
export class Navigation {
greeting() {
return name()
.then(name => {
return name ?
`Welcome Back, ${name}!` :
'Welcome!';
});
}
};
Navigation Test Code:
import QUnit from 'qunit';
import clone from 'steal-clone';
let navigation, name;
QUnit.module('navigation', {
beforeEach(assert) {
const done = assert.async();
clone({
'models/user': {
name() {
return Promise.resolve(name);
}
}
})
.import('navigation')
.then(({ Navigation }) => {
navigation = new Navigation();
done();
});
}
});
QUnit.test('greeting - no name', (assert) => {
const done = assert.async();
name = null;
navigation
.greeting()
.then(greeting => {
QUnit.equal(greeting, 'Welcome!');
done();
});
});
QUnit.test('greeting - with name', (assert) => {
const done = assert.async();
name = 'Kevin';
navigation
.greeting()
.then(greeting => {
QUnit.equal(greeting, 'Welcome Back, Kevin!');
done();
});
});
You’ll notice a few things in this code:
- This example is using ES6 module syntax, but if you prefer CommonJS or AMD that is fine too; steal-clone will work with whatever syntax you choose.
- You only use Dependency Injection exactly where you want it. You’re not forced to use any libraries or frameworks in your modules.
This simple example shows how Dependency Injection can isolate your tests to exactly the module you’re testing and how simple it is to use DI with StealJS and steal-clone. If you want to start simplifying your tests and you’re already using StealJS, take a look at the docs for steal-clone and start using it today. If you’re not using StealJS, take a look at its homepage and docs to get started.
Kevin Phillips
Director of Backend Development